728x90
스프링부트에서 정적 파일인 이미지를 어떻게 보여주는지 다시한번 구글링해보았다.
여러가지 방법이 있었던것 같은데 나는 정적인 이미지 파일이니까 해당 파일이 있는 위치에서 바로 불러오고 싶었다.
그래서 한번 찾아보았다..
@GetMapping("/{imageName}")
public ResponseEntity<byte[]> getImage(@PathVariable String imageName) throws IOException {
// 여기서는 예를 들어 images 디렉토리에서 이미지를 로드합니다
Resource imgFile = new ClassPathResource("static/images/" + imageName);
InputStream in = imgFile.getInputStream();
byte[] imageBytes = StreamUtils.copyToByteArray(in);
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.IMAGE_JPEG); // 이미지 타입에 따라 MediaType 설정
headers.setContentLength(imageBytes.length);
return new ResponseEntity<>(imageBytes, headers, HttpStatus.OK);
}
이 코드를 사용하면 바로 해당 위치의 파일 바이트를 복사해서 보여주는 기능을 할 수 있다.!!
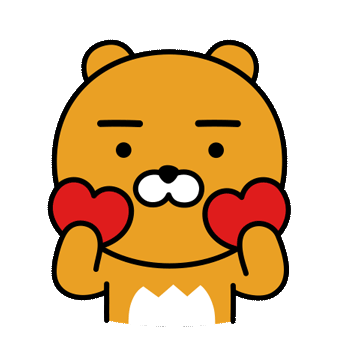
'Springboot' 카테고리의 다른 글
Centos 7 jar 파일 스프링부트 프로퍼티 구분하기 (2) | 2024.10.14 |
---|---|
Springboot_ import 오류 (1) | 2024.09.18 |
Springboot_ mybatis type-aliases 설정 (0) | 2024.08.21 |
Springboot_ 실행하자마자 종료되는 이유 (0) | 2024.08.20 |
Springboot_ jdbc 연동 (0) | 2024.08.20 |